Table of Contents
Introduction
Communication between Android applications and servers is a critical aspect of application development, and choosing the right tool to perform this communication is fundamental. Until now, Retrofit has been the standard for excellence.
However, in recent years an interesting alternative has emerged: Ktor Client.
This library, developed by JetBrains entirely in Kotlin, offers a more modern and flexible way to make HTTP requests without relying on annotations, with a simpler and easier-to-understand syntax. Its plugins, such as authentication and serialization exist as external dependencies, so you can install only the ones you need, making it a lightweight library. It is also cross-platform.
In this article, we will see practical examples that compare both clients and provide an overview for migrating any project. Please note: we assume the reader has basic knowledge of Retrofit, so we will not go into the details of the library.
Base Case
Let’s imagine the scenario where we have this basic Retrofit setup: we use Gson Converter, wrap the server response in Kotlin Result and log the HTTP interaction using an interceptor.
implementation "com.squareup.retrofit2:retrofit:$retrofit_version"
implementation "com.squareup.retrofit2:converter-gson:$retrofit_version"
implementation "com.github.skydoves:retrofit-adapters-result:$retrofit_result_version" implementation "com.squareup.okhttp3:logging-interceptor:$logging_interceptor_version"
// creates okHttp engine with logger interceptor
val okHttp = OkHttpClient.Builder()
.addInterceptor(HttpLoggingInterceptor()
.apply {
setLevel(
HttpLoggingInterceptor.Level.BODY
)
})
.build()
val retrofit = Retrofit.Builder()
// add base url for all request
.baseUrl(BASE_URL)
// add gson content negotiation
.addConverterFactory(GsonConverterFactory.create())
// add adapter to wrap response with result
.addCallAdapterFactory(ResultCallAdapterFactory.create())
.client(okHttp)
.build()
How to set up Ktor
To migrate to Ktor we would need these dependencies:
implementation "io.ktor:ktor-client-core:$ktor_version"
implementation "io.ktor:ktor-client-okhttp:$ktor_version"
implementation "io.ktor:ktor-client-content-negotiation:$ktor_version"
implementation "io.ktor:ktor-serialization-gson:$ktor_version"
implementation "com.squareup.okhttp3:logging-interceptor:$logging_interceptor_version"
ktor-client-core
Ktor’s main library includes interesting plugins such as HttpRequestRetry, HttpSend, and HttpCache, among others.ktor-client-okhttp
engine to process network requests. There are several android compatible engines: OkHttp (used in Retrofit, supports Http/2 and WebSockets), CIO (coroutine-based implementation), and Android (an engine that supports older versions of Android 1.x+)ktor-client-content-negotiation
plugin to serialize/deserialize content.ktor-serialization-gson
JSON serializer. There are other official alternatives such as Jackson and Kotlin’s serialization.
Once the libraries have been added the equivalent Ktor configuration would be:
// creates Ktor client with OkHttp engine
val Ktor = HttpClient(OkHttp) {
// default validation to throw exceptions for non-2xx responses
expectSuccess = true
engine {
// add logging interceptor
addInterceptor(HttpLoggingInterceptor().apply {
setLevel(
HttpLoggingInterceptor.Level.BODY
)
})
}
// set default request parameters
defaultRequest {
// add base url for all request
url(BASE_URL)
}
// use gson content negotiation for serialize or deserialize
install(ContentNegotiation) {
gson()
}
}
Using the OkHttp engine allows us to reuse the interceptors we have created in our project.
How to create the ApiService
In Retrofit, we define an interface and each method must have an HTTP annotation that provides the request method and the library is in charge of generating the code at compile time. While Ktor provides equivalent functions to these annotations and we can reuse the existing interface (removing the retrofit annotations) so as not to break the contract, we are in charge of defining the implementation of these requests.
// Retrofit create a service
val service = retrofit.create<UserApiService>()
—-------—-------—-------—-------—-------—-------—-------—-------
// Ktor create a service
val service: UserApiService = UserApiServiceImpl(Ktor)
How to make requests
Now that we have the client configured let’s see some examples of how to make a GET request, how to manipulate the URL by replacing blocks with Path, and how to add Query or Header parameters, along with the different ways to send data.
GET requests – URL Manipulation
- Retrofit
interface UserApiService {
@GET("/user")
suspend fun getUsers(): Result<Users>
// Replacements blocks
@GET("/user/{id}")
suspend fun getUserById(@Path("id") id: Int): Result<User>
// Query parameter
@GET("/user")
suspend fun getUsers(@Query("page") page: Int): Result<Users>
// Complex query combinations
@GET("/user")
suspend fun getUsers(@QueryMap queries: Map<String, String>): Result<Users>
}
- Ktor
class UserApiServiceImpl(private val Ktor: HttpClient) : UserApiService {
suspend fun getUsers(): Result<Users> = runCatching {
Ktor.get("/user").body()
}
// Replacements blocks
suspend fun getUserById(id: Int): Result<User> = runCatching {
Ktor.get("/user/$id").body()
}
// Query parameters
suspend fun getUsers(page: Int): Result<Users> = runCatching {
Ktor.get("/user") {
parameter("page", page)
}.body()
}
// Complex query combinations
suspend fun getUsers(queries: Map<String, String>): Result<Users> = runCatching {
Ktor.get("/user") {
queries.forEach {
parameter(it.key, it.value)
}
}.body()
}
}
POST requests – Sending data
- Retrofit
// request body
@POST("/user")
suspend fun createUser(@Body user: User): Result<User>
// request using x-www-form-urlencoded
@FormUrlEncoded
@POST("/user/edit")
suspend fun editUser(
@Field("first_name") first: String,
@Field("last_name") last: String
): Result<User>
// request multi-part
@Multipart
@POST("user/update")
suspend fun updateUser(@Part("photo") photo: MultipartBody.Part): Result<User>
- Ktor
// request body
suspend fun createUser(user: User): Result<User> = runCatching {
Ktor.post("/user") { setBody(user) }.body()
}
// request using x-www-form-urlencoded
suspend fun editUser(first: String, last: String): Result<User> = runCatching {
Ktor.submitForm(
url = "/user/edit",
formParameters = Parameters.build {
append("first_name", first)
append("last_name", last)
}
).body()
}
// request multi-part
suspend fun updateUser(photo: String): Result<User> = runCatching {
Ktor.post("/user/update") {
setBody(MultiPartFormDataContent(
formData {
append("photo", File(photo).readBytes())
}
))
}.body()
}
Headers
- Retrofit
@Headers("Cache-Control: max-age=640000")
@GET("/user")
suspend fun getUsers(): Result<Users>
@Headers({
"Accept: application/vnd.github.v3.full+json",
"User-Agent: Retrofit-App"
})
@GET("/user")
suspend fun getUsers(): Result<Users>
@GET("/user")
suspend fun getUsers(@Header("Authorization") auth: String): Result<Users>
- Ktor
suspend fun getUsers(): Result<Users> = runCatching {
Ktor.get("/user") {
headers {
append("header", "value")
append("header", "value1") // this value will be appended to previous
appendIfNameAbsent("header", "value2") // this won't be added as name exists
appendIfNameAndValueAbsent("header2", "value1") // this won't be added
}
}.body()
}
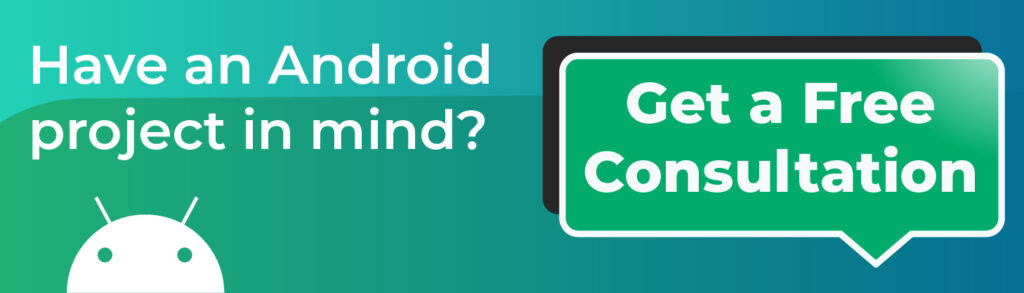
Conclusion
In short, the Ktor Client implementation is a bit more verbose but at the same time, it is easy to understand and highly configurable. For example, we can type the URL, which by having the requests in a structured way facilitates the maintenance, while providing greater control/security over the types used. It also allows us to create custom plugins that among other things make it easier to intercept calls.
And yes, it is a bit cumbersome to wrap the call each time in a runCatching
or do .body()
to get the payload of the response but with this little extension, we avoid repeating ourselves.
suspend inline fun <reified R> HttpClient.getResult(
urlString: String,
builder: HttpRequestBuilder.() -> Unit = {}
): Result<R> = runCatching { get(urlString, builder).body() }
Interested in learning more about different Android development topics? I suggest you take a look at Apiumhub‘s blog. There you will find articles on the latest mobile technologies as well as in frontend development, backend development and software architecture.
Author
-
Android Passionate Developer that enjoy solving issues. Focused on developing quality and scalable software.
View all posts