Table of Contents
Introduction: Gradle version catalogs
Gradle version catalogs allow us to add and maintain dependencies in an easy and scalable way. Apps grow, and managing projects with several development teams increase the compilation time.
To address this issue, one potential solution involves segmenting the project into multiple modules. Compiling these modules in parallel and solely recompiling modified portions reduces the overall compilation time. However, a predicament arises: how can we effectively share common library dependencies and their respective versions, while evading compilation errors and the necessity to manually scrutinize Gradle files in each module to prevent synchronization issues?
Gradle version catalogs help us centralize all the dependencies in a single file with safe typing that you can call from your modules.
Create version file
First, we have to create our file in the Gradle folder. By convention, we use the name libs.version.toml
recommended from the Android development guides.
This file will have three sections:
[versions]
[libraries]
[plugins]
- Versions. It defines the versions of your dependencies and plugins that are used in the other two blocks
- Libraries. Contains the dependencies.
- Plugins. Contains the plugins.
Let’s define as an example the version and dependencies of the Koin Dependency Injection library.
[versions]
koin = "3.4.0"
[libraries]
koin-core = { group = "io.insert-koin", name = "koin-core", version.ref = "koin" }
koin-android = { group = "io.insert-koin", name = "koin-android", version.ref = "koin" }
koin-androidx-compose = { group = "io.insert-koin", name = "koin-androidx-compose", version.ref = "koin" }
koin-androidx-workmanager = { group = "io.insert-koin", name = "koin-androidx-workmanager", version.ref = "koin" }
koin-test = { group = "io.insert-koin", name = "koin-test", version.ref = "koin" }
koin-test-junit4 = { group = "io.insert-koin", name = "koin-test-junit4", version.ref = "koin" }
Then to call the dependency from any module we would only have to do:
Dependencies {
implementation(libs.koin.core)
implementation(libs.koin.android)
}
Create your plugins and all your modules will always be in sync
When we already have our dependencies migrated, we see a problem. All our modules that need Koin will have to repeat the same lines. Here we can see a clear example of DRY (Don’t Repeat Yourself), and we will create a Gradle plugin to save us from repeating, again and again, the inclusion of these dependencies.
In our build.gradle.kts
file we will be able to register our gradle plugins
gradlePlugin {
plugins {
register("koin") {
id = "example.android.koin"
implementationClass = "AndroidKoinConventionPlugin"
}
}
}
Then we will create our plugin where we will add the dependencies of this plugin with GVC.
class AndroidKoinConventionPlugin : Plugin<Project> {
override fun apply(target: Project) {
with(target) {
val libs = extensions.getByType<VersionCatalogsExtension>().named("libs")
dependencies {
"implementation"(libs.findLibrary("koin.core").get())
"implementation"(libs.findLibrary("koin.android").get())
"implementation"(libs.findLibrary("koin.androidx.compose").get())
"implementation"(libs.findLibrary("koin.androidx.workmanager").get())
"testImplementation"(libs.findLibrary("koin.test").get())
"testImplementation"(libs.findLibrary("koin.test.junit4").get())
}
}
}
}
The only thing left is to call in our modules to the Koin plugin, and if someday, we have to upgrade the version, we only have to go to our definitions file, and all of them will be updated when synchronizing gradle. For example, the data module file with all its dependencies:
plugins {
id("example.android.library")
id("example.android.koin")
}
android {
namespace = "com.example.core.data"
}
dependencies {
implementation(libs.kotlinx.coroutines.android)
}
With all this, we can create plugins that group dependencies, for example:
- Library
- Compose
- Flavors
- Feature
- DI
- Database
- Test
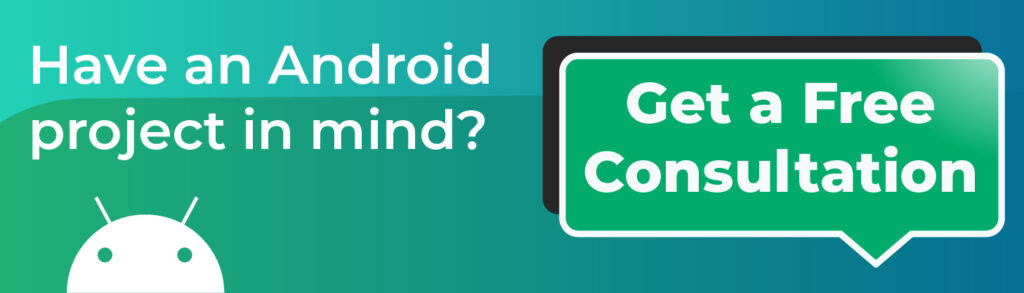
Considerations
Gradle version catalogs are still in full development, and it is good to follow the known bugs and limitations that Android is publishing. The examples in this article show the benefits of Gradle version catalogs and how they can make our lives easier. If you are looking for a functional application of it, you can see it on GitHub now for Android.
Are you interested in reading more about Android development? I suggest you visit Apiumhub’s blog to stay up-to-date with the latest technologies. Every week, new and exciting content about Android development, software architecture, frontend development, backend development, and more is published.