Table of Contents
Based on the book Atomic Design by Brad Frost.
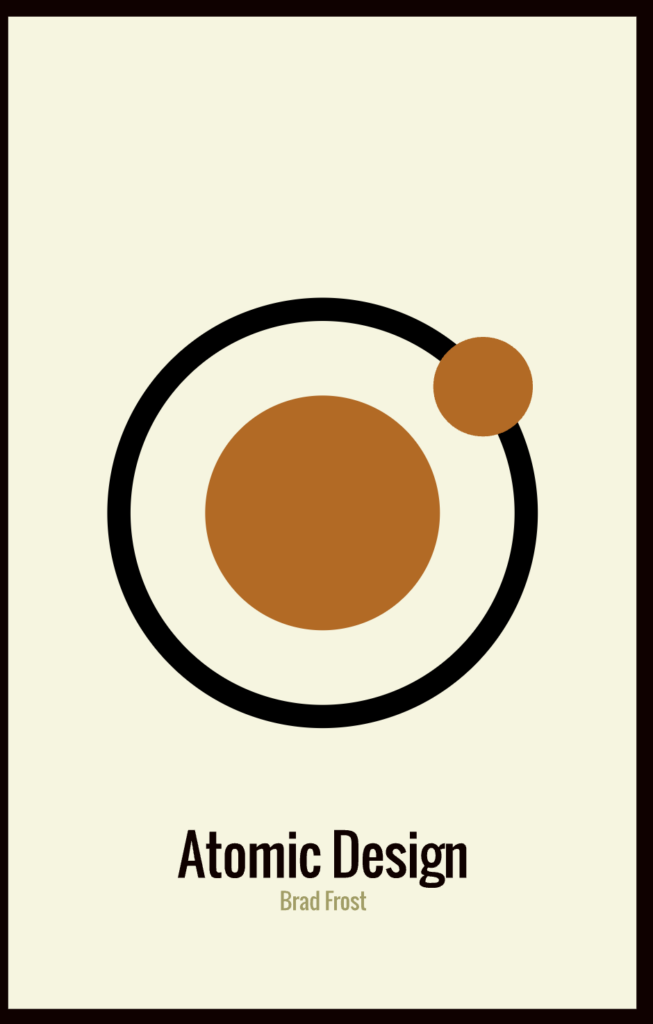
What is Atomic Design?
Atomic Design is a methodology for designing and developing user interfaces that have gained popularity in recent times. It was proposed by Brad Frost as a systematic approach to creating reusable and consistent components in web applications, following an analogy with the structure of atoms and molecules in chemistry.
This approach organizes the elements of an interface in hierarchical levels, from the most basic and simple components to the most complex ones.
In this article, we will explore how to apply Atomic Design in a VueJS project, one of the most popular frameworks for building web applications.
Benefits
Scalability and Consistency: Atomic Design’s modular approach facilitates the creation of reusable components. This makes it possible to build scalable interfaces that are easy to maintain since changes made to a component can be propagated to all instances where it is used.
Scalability: By dividing the interface into hierarchical components, Atomic Design allows for a scalable architecture. Atoms and molecules can be combined to build more complex organisms and, in turn, templates and pages. This facilitates the process of adding new features and functionality as the application grows and evolves.
Facilitates Teamwork: By following a clear structure and nomenclature for components, Atomic Design facilitates collaboration between designers and developers. All team members share a common vocabulary and understanding of how the different interface elements are constructed and composed.
Speed of Development: Atomic Design’s component reuse and organized structure accelerate the development process. Teams can build interfaces faster by using already designed and tested components.
Facilitates Responsive Design: Atomic Design encourages the creation of components that easily adapt to different screen sizes and devices. Atoms and molecules can be individually designed and tested at different resolutions, which facilitates the creation of responsive and adaptive interfaces.
Clear and Complete Documentation: By having a well-defined component hierarchy, Atomic Design documentation becomes more structured and complete. Teams can create component libraries and maintain a consistent style guide, resulting in more useful and accessible documentation for future developments and new team members.
Improved Maintainability: By having a clear and organized structure, maintenance and refactoring of the interface becomes easier. Changes to an atomic component will affect only the instances where it is used, which reduces the risk of introducing errors in other parts of the application.
Encourages Creativity and Experimentation: While Atomic Design establishes structure, it also allows for creativity and experimentation within components. Designers and developers can try different combinations of atoms and molecules to achieve new solutions and innovative experiences.
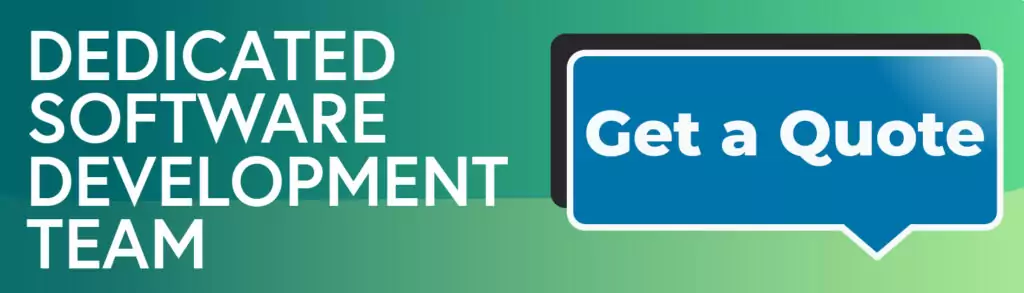
Implementing Atomic Design with Vue.js
Vue.js is a progressive framework that facilitates the creation of reusable components. Let’s see how we can apply Atomic Design in Vue.js.
1.Atoms
<template>
<button class="btn" @click="onClick">
<slot></slot>
</button>
</template>
<script>
export default {
methods: {
onClick() {
this.$emit('click');
}
}
}
</script>
2. Molecules
Molecules use atoms, in this case, we will create a SearchInput.
<template>
<div class="search-input">
<input type="text" class="search-input__field" placeholder="Buscar" v-model="query" />
<Button @click="onSearch">Buscar</Button>
</div>
</template>
<script>
import Button from './Button';
export default {
components: {
Button
},
data() {
return {
query: ''
};
},
methods: {
onSearch() {
// Lógica de búsqueda aquí
}
}
}
</script>
3. Organisms
Following the Atomic Design approach, we can create an organism that groups the molecule SearchInput along with other related components, such as a registration form.
<template>
<form class="registration-form">
<h2>Formulario de Registro</h2>
<SearchInput />
<Button @click="onSubmit">Registrarse</Button>
</form>
</template>
<script>
import SearchInput from './SearchInput';
export default {
components: {
SearchInput
},
methods: {
onSubmit() {
// Lógica de envío del formulario aquí
}
}
}
</script>
4. Templates and Pages
The next level in Atomic Design is templates and pages. Templates define the overall structure and the overall structure and design, while pages are specific instances of those templates. templates.
<!-- Template -->
<template>
<div class="app">
<header>
<slot name="header"></slot>
</header>
<main>
<slot></slot>
</main>
<footer>
<slot name="footer"></slot>
</footer>
</div>
</template>
<!-- Page -->
<template>
<App>
<template v-slot:header>
<h1>Mi Aplicación Vue</h1>
</template>
<RegistrationForm />
<!-- Otras instancias de componentes aquí -->
<template v-slot:footer>
<p>Derechos de autor 2023 - Mi Aplicación Vue</p>
</template>
</App>
</template>
The illustration can be seen below to help assimilate the above examples.
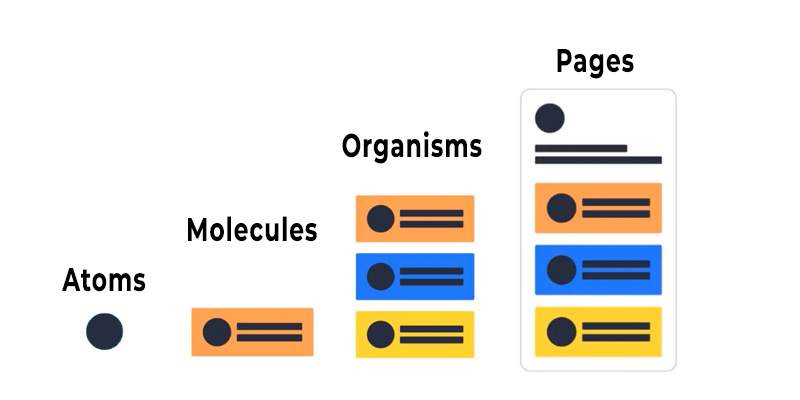
Directory organization of a Vue.js project using Atomic Design
In this scheme, atomic components, molecules and organisms are grouped inside the components folder. Templates are located in the layouts folder, and pages are located in the pages folder.
Remember that this structure may vary according to the size and complexity of the project, but by following this organization, you will maintain a clear and modular structure that will facilitate collaboration and maintenance throughout the development of the project.
src/
|-- assets/
|-- components/
| |-- atoms/
| | |-- Button.vue
| | |-- Icon.vue
| | |-- ...
| |
| |-- molecules/
| | |-- SearchInput.vue
| | |-- ...
| |
| |-- organisms/
| | |-- Header.vue
| | |-- Form.vue
| | |-- ...
|
|-- layouts/
| |-- DefaultLayout.vue
| |-- ...
|
|-- pages/
| |-- HomePage.vue
| |-- AboutPage.vue
| |-- ...
|
|-- router/
| |-- index.js
|
|-- store/
| |-- index.js
| |-- modules/
| | |-- ...
|
|-- App.vue
|-- main.js
When should we use Atomic Design?
In the book “Atomic Design” by Brad Frost, it is suggested to use the Atomic Design methodology in the context of designing and developing user interfaces for web and mobile applications. The methodology can be applied in a variety of scenarios, and Brad Frost provides some specific situations where Atomic Design is especially useful:
Large-Scale Projects: In large-scale projects, where the interface contains numerous components and functionalities, Atomic Design is an effective way to maintain consistency and organization. The methodology facilitates the creation and management of reusable components, which reduces code duplication and improves the maintainability of the project as it grows.
Multidisciplinary Teams: Atomic Design is especially valuable when working in multidisciplinary teams that include designers, developers, and other specialists. The clear, hierarchical structure of the methodology facilitates collaboration and communication among team members.
Projects Requiring Flexibility: In projects where experimentation and frequent changes are necessary, Atomic Design Atomic Design offers a flexible foundation. Modular components allow you to iterate quickly and test different combinations to find the best solution. find the best solution.
Responsive and Adaptive Design: Atomic Design is suitable for projects that need to be compatible with different screen sizes and devices. The hierarchical and modular The hierarchical and modular structure of the methodology facilitates the creation of components that adapt to different contexts.
Creation of Design Systems: Atomic Design is especially useful for developing consistent and scalable design systems. By organizing components into atoms, molecules, and organisms, a library of reusable components is created that can be used throughout the interface.
Long-term projects: For projects that require long-term maintenance, Atomic Design provides an organized structure that facilitates the addition of new team members and ongoing code management.
Conclusion
Overall, Atomic Design is a versatile methodology that can be applied to a variety of projects, but it especially excels in large, collaborative projects that seek to maintain a consistent and reusable interface. By following the methodology, teams can optimize their workflow and develop more efficient and robust user interfaces.
Atomic Design is a powerful methodology for developing scalable and reusable user interfaces. In Vue.js, we can apply these principles to build atomic components, molecules, organisms, templates, and pages. By following this organized structure, our code will be more maintainable, understandable, and easy to extend. Vue.js offers an incredibly flexible and powerful ecosystem for implementing Atomic Design, allowing developers to create sophisticated and consistent web applications.
Author
-
Software developer with over 7 years experience working with several programming languages and frameworks. Passionate about learning something new every day
View all posts